In this post we’ll be doing an overview of lifecycle hooks in Vue.js.
Each Vue application first creates a Vue instance, with the Vue function:
var vm = new Vue({
router,
render: function (createElement) {
return createElement(App)
}
}).$mount('#app')
This Vue instance during its initialization goes through several phases and it exposes some properties and methods in each phase. This is an example of Vue using the template method behavioural design pattern.
The methods which run by default in this process of creating and updating the DOM are called lifecycle hooks and using them properly allows easy access to a behind the scenes overview of how the library works.
Below we can see a simple diagram showcasing all methods in one instance.
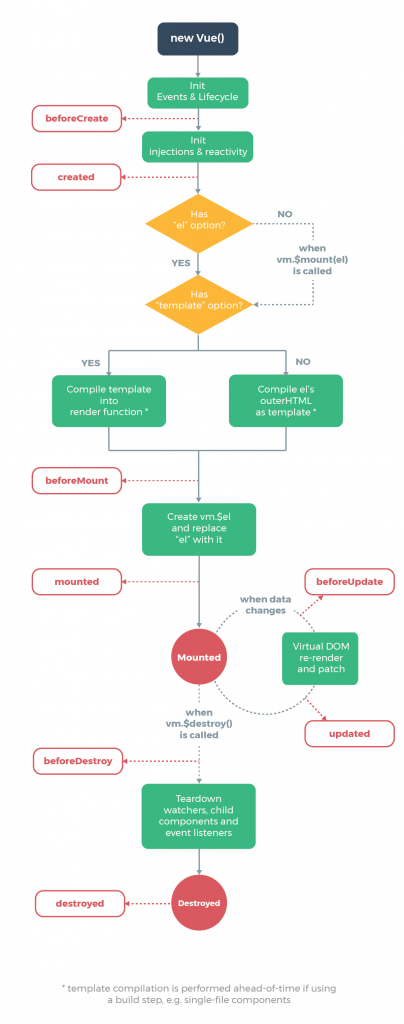
We can see that we have 8 methods that we can split into 4 phases in an application’s lifecycle.
- Creation or initialization hooks (beforeCreate, created)
- Mounting or DOM insertion hooks (beforeMount, mounted)
- Updating hooks (beforeUpdate, updated)
- Destroying hooks (beforeDestroy, destroyed)
Creation hooks
beforeCreate is the first hook that gets called in a Vue component, it has no access to the components reactive data and events as they haven’t been initialized yet. It’s good to use this hook for non-reactive data.
The created hook has access to the components events and reactive data and state. The DOM and $el properties are still not available. This hook is usually used to perform API calls and store the response.
Mounting hooks
The next lifecycle hook to be called is beforeMount and it happens right before the component is mounted on the DOM. Here is our last opportunity to perform operations before the DOM gets rendered.
Mounted is called right after and now the DOM is available. This is a good place to add third-party integrations like charts, Google Maps etc, which interact directly with the DOM.
mounted() {
console.log('This is the DOM instance', this.$el)
this.$nextTick(function() {
console.log('Child components have now been loaded')
}
}
Updating hooks
beforeUpdate and updated are the two hooks that are called each time a reactive property is changed. The data in beforeUpdate holds the previous values of the property, while after updated runs, the instance has finished re-rendering.
beforeUpdate () {
console.log('before update called')
},
updated () {
console.log('update finished')
}
Destroying hooks
When beforeDestroy, we can still mutate the state and the instance is still functional. Here we can do some last moment data mutation before the instance is destroyed.
When destroy is called, the Vue instance does not exist anymore. All directives, event listeners have been removed and child components have been destroyed.
beforeDestroy () {
console.log('before destroy called')
},
destroyed () {
console.log('destroyed')
}
I hope this provides a good overview of the lifecycle hooks in a Vue application. For more information, refer to the official docs here.