One of the most important things when doing test automation is effectively reporting the test results from our executions. This allows us to faster detect issues in the applications, reporting those issues and mitigating them. After each test run cypress provides us with screenshots, videos and test files that depend on the reporter that was selected in the config file. In this article, I will show you how to generate mochawesome html report and enabling multiple reporting, using the cypress-multi-reporters plugin.
HTML – Mochawesome report
The first step is to enable reporting in the cypress.config file. The following properties should be set as follows:
reporter: "mochawesome",
reporterOptions: {
reportDir: "tests/results",
overwrite: false,
json: true,
},
- “reporter” property sets the reporter that will be used.
- “reportDir” property sets the path where the test results will be stored.
- “json” property is set to true, to create json test result files.
With the following properties set, after test execution the json files will be created in the specified path. Each of those files corresponds to one file with extension “cy” (old naming “spec”) file in our project.
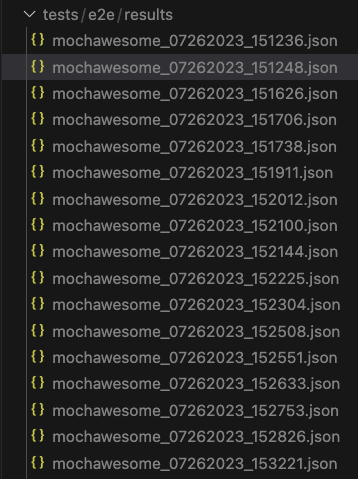
Next step is to merge all those files into single .json file (called report.json) that will contain the test results of all tests, so further on we can use to create our html report. For that purpose, the following merge-reports.js file is created:
const { merge } = require("mochawesome-merge");
const mergeFile = require("mochawesome-report-generator");
const cypressConfig = require("./cypress.json");
var fs = require("fs");
const reportDir = cypressConfig.reporterOptions.reportDir;
console.log("reportDir is", reportDir);
const mergeOptions = {
files: [`./${reportDir}/*.json`],
output: `./${reportDir}/report.json`,
};
return merge(mergeOptions).then((report) => {
console.log("time to call merge on", report);
fs.writeFile("./results/report.json", JSON.stringify(report), (err) => {
if (err) {
console.error(err);
return;
}
console.log("File has been created");
});
mergeFile.create(report, {
reportDir,
reportFilename: "Name of html file",
reportTitle: "Name of the report.",
reportPageTitle: "Title of the report",
});
});
After executing the merge_reports.js file, the html report is created in the results folder. Opening the file should display descriptive report of our last test run, that will look like this:
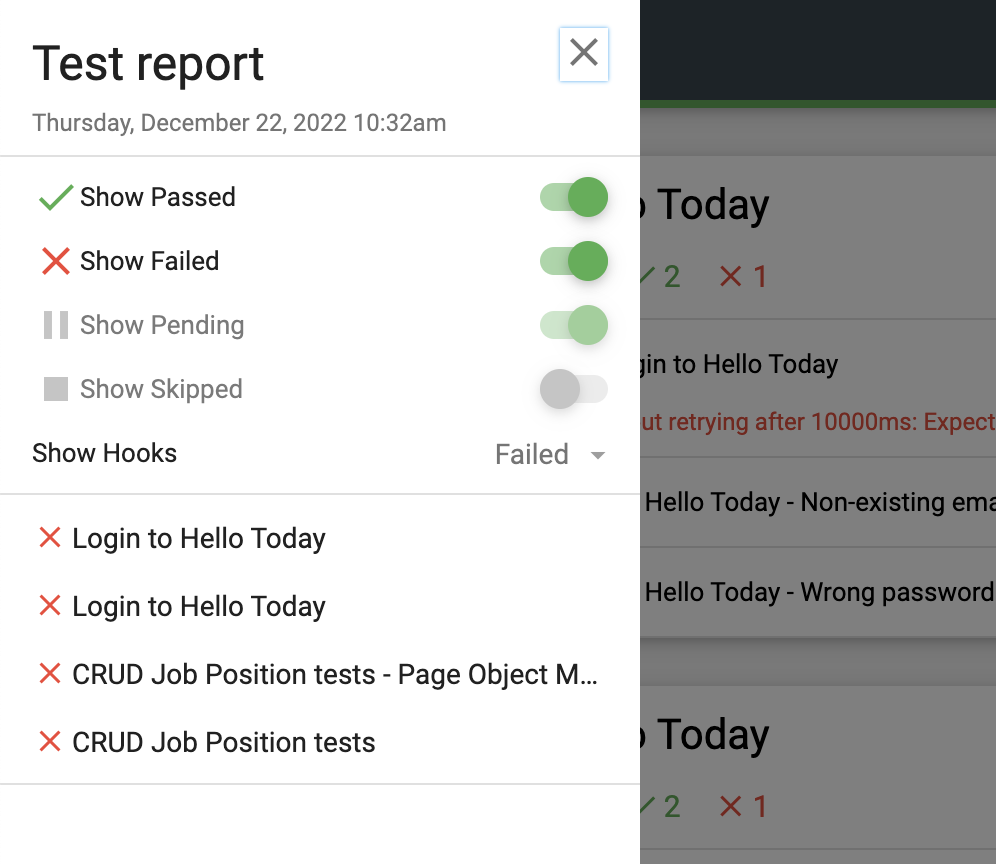
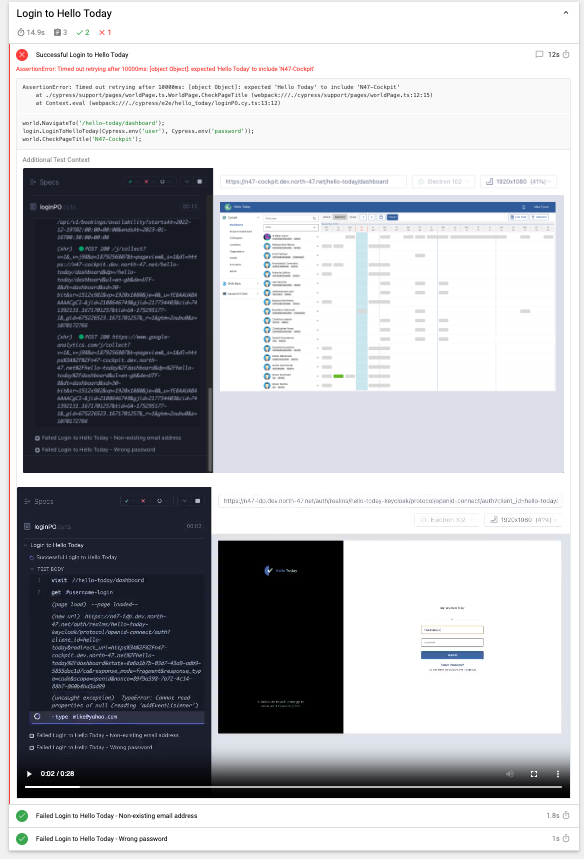
The page has a menu with which the tests can be filtered by status. Each failed tests also includes screenshots, videos and the error with which the test failed. All this can be used to quickly create descriptive bugs.
Multiple reporting in Cypress
In order to create different type of reports for the same project, different type of result files are needed. To generate them, first cypress-multi-reporter extension needs to be installed with following command:
npm install cypress-multi-reporters --save-dev
After installing the extension a separate reporterConfig.js file needs to be added to the project. This file will defines which reporters are used when running the cypress tests and also their preferences. “reporterEnabled” parameter defines which reporters will be used. (In the example mochawesome and mocha-junit-reporter are used). The file would look like as follows:
module.exports = {
reporterEnabled: "mochawesome, mocha-junit-reporter",
mochawesomeReporterOptions: {
reportDir: "tests/e2e/results",
overwrite: false,
json: true,
},
mochaJunitReporterReporterOptions: {
mochaFile: "tests/e2e/results/test-results-[hash].xml",
testCaseSwitchClassnameAndName: false,
},
};
To activate the multi reporting when running the tests, the following parameters need to be passed in the command:
--reporter cypress-multi-reporters --reporter-options configFile=reporterConfig.js
When the test execution ends, for each cy/spec test file a json and xml results file is created. These files, as previously demonstrated when creating the html report, can be used to created different kind of reports.
Conclusion
Reporting is essential for good automated tests setup and cypress allows us to create very detail reports with all the necessary information. This eases out any QA/DEVs life and lowers the time needed for creating bugs, raising issues for the web applications. These reports can also be used to report results in xRay, TestRail and many other test reporting tools.