Overview
Camunda BPM is a light-weight, open-source platform for Business Process Management. It ships with tools for creating workflow and decision models, operating deployed models in production, and allowing users to execute workflow tasks assigned to them. It is developed in Java and released as open-source software under the terms of Apache License.
Modeling your first process
In order to show how Camunda works and looks like I will use this simple process. Let us imagine that you want to introduce a review process on your Twitter account and have every tweet go through this review process.
One way to manage this is to make a web application from scratch for this scenario. But we can also model this process with Camunda Modeler and utilize Camunda for this workflow.
On the following image, it is shown one way to model this process with standard BPMN model using Camunda Modeler:
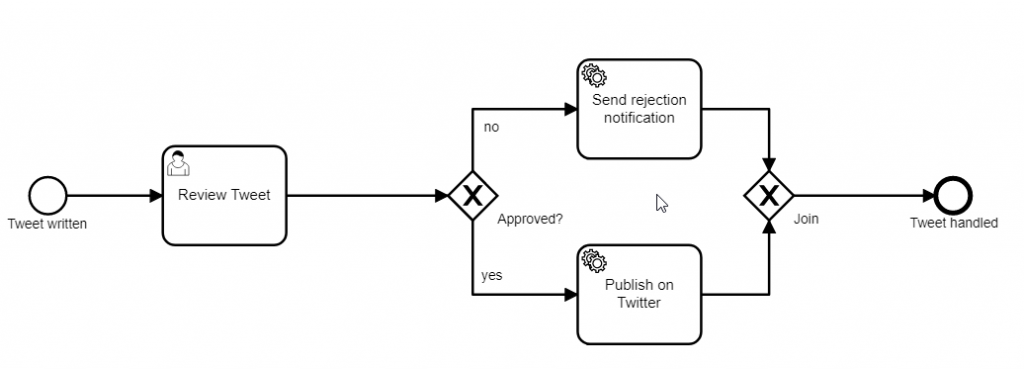
In this diagram, the process is started when someone writes a new tweet. After that, we have a human task where someone has to review this tweet and decide its approval status. And after that we have two possible options if the tweet is approved, a service task is called that will publish this on Twitter. If rejected we again call a service task, however this time we notify the user that his tweet was rejected.
I will go through all of these steps in more detail.
Starting the process
Camunda processes can be started programmatically using some of their supported SDKs like Java or by using the Camunda Tasklist GUI that comes out of the box. In this case, I will use the Camunda tasklist to start a new tweet.
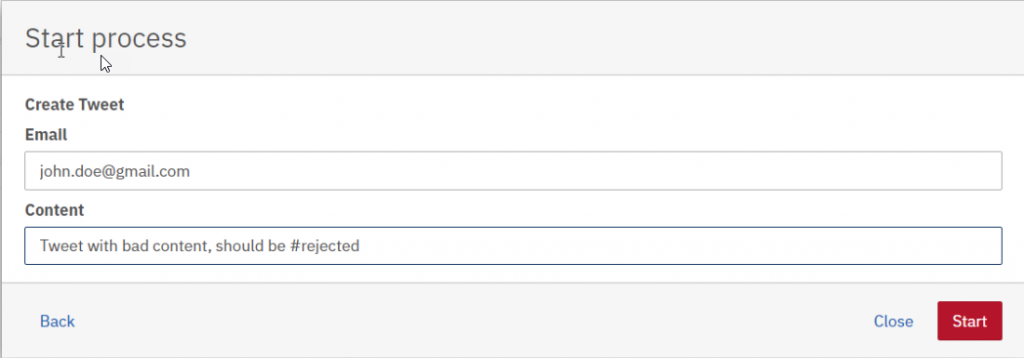
Working on the human task
Human tasks are tasks that must be manually completed by some users. And this can be something as simple as completing a form or it can be something like actually shipping an item somewhere. They are visible in the Camunda Tasklist GUI and users can assign a certain task to themselves and complete them.
In our Camunda BPMN model, the next step in the process is a human task. In our process, we want to review the tweet in this task. On the following image is shown how the human tasks look like by default in Camunda Tasklist:
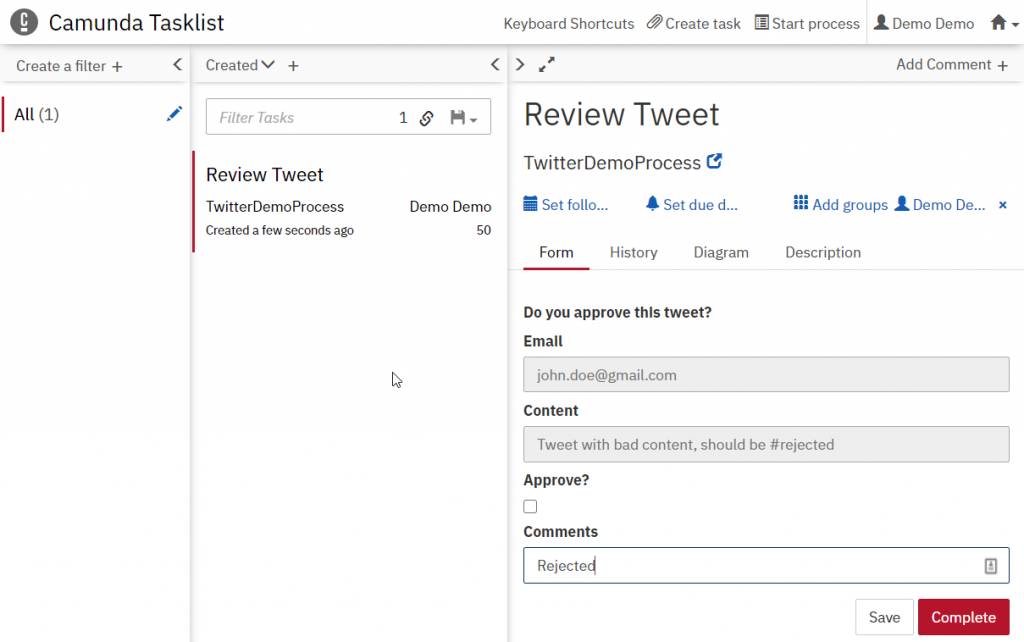
Automating service tasks
Service task is used to invoke some service, this can be some Java code or some asynchronous external worker.
After the tweet is reviewed we have ‘conditional flow’ in Camunda, which depends on whatever the tweet was approved or not, decides how the flow should continue. In both cases, our flow continues with a service task.
In our case, we have two separate service tasks. One is called when a tweet is rejected and will send a notification, while the other one is used when the tweet is approved and will publish it on Twitter.
First, let us take a look at the service tasks for sending rejection notification:
@Slf4j
@Service("emailAdapter")
public class RejectionNotificationDelegate implements JavaDelegate {
public void execute(DelegateExecution execution) throws Exception {
String content = (String) execution.getVariable("content");
String comments = (String) execution.getVariable("comments");
log.info("Hi!\n\n"
+ "Unfortunately your tweet has been rejected.\n\n"
+ "Original content: {}\n\n"
+ "Comment: {}\n\n"
+ "Sorry, please try with better content the next time :-)", content, comments);
}
}
In this code, we obtain process variables like tweet content and rejection comments and we log them in the console. This logic, of course, can be extended to send actual emails, the important thing here is that in order to model Camunda service we only need to implement JavaDelegate
interface and override execute
method.
In the next code snippet, we have the snippet for publishing the tweet:
public class TweetContentDelegate implements JavaDelegate {
public void execute(DelegateExecution execution) throws Exception {
String content = (String) execution.getVariable("content");
AccessToken accessToken = new AccessToken("token", "secret");
Twitter twitter = new TwitterFactory().getInstance();
twitter.setOAuthConsumer("consumer");
twitter.setOAuthAccessToken(accessToken);
twitter.updateStatus(content);
}
}
As in the previous code, we also have to implement JavaDelegate
and override execute
method.
More Camunda examples can be found on their official GitHub repository: https://github.com/camunda/camunda-bpm-examples
Conclusion
In the above diagram, we have only seen one example of a process, but Camunda offers a lot more features for modeling business processes and a lot of out-of-the-box implementations that save a lot of time. Also, almost everything is customizable.
If your company has a lot of processes that can be modeled as a BPMN process or processes that require human intervention then Camunda can be the right tool for the job.
In my opinion, it’s definitely worth to have a basic understanding of how Camunda works in order to be able to spot a use-case for this tool.