Arrow functions have been adopted since ECMAScript 2015. They are very powerful and simple. Many ES5-based projects adopted this feature to refactor the code. However, the arrow functions aren’t the same as the normal functions you’ve got to know. In this blog, I will explain what is the difference between normal and arrow functions.
This Keyword
A normal functions .this – binding is determined by who calls the function:
let a = 10;
let obj = {
hello,
a: 20
};
function hello() {
console.log(this.a);
}
hello(); // outputs 10
obj.hello(); //outputs 20
The method hello gives a different result by how it’s called. This is because a normal functions this is bound to the object that calls the function.
In contrast to a normal function, an arrow functions this is always bound to the outer function that surrounds the inner function:
let a = 10;
let hello = () => {
console.log(this.a);
};
let obj = {
hello,
a: 20
}
hello(); // outputs 10
obj.hello(); //outputs 10
In this example, hello is an arrow function. It’s a property of the obj. Even though obj calls hello, it still prints 10 because a function’ this always refers to the outer environments this. And the global this is a window so it points out to window.x.
Arguments
A normal function has a special property called argument:
function hello () {
console.log(arguments.length)
};
hello(1, 2, 3); // outputs 3
hello(10); // outputs 1
An arrow function doesn’t have an arguments property:
let hello = () => {
console.log(arguments.length)
};
hello(1, 2, 3); // outputs arguments is not defined
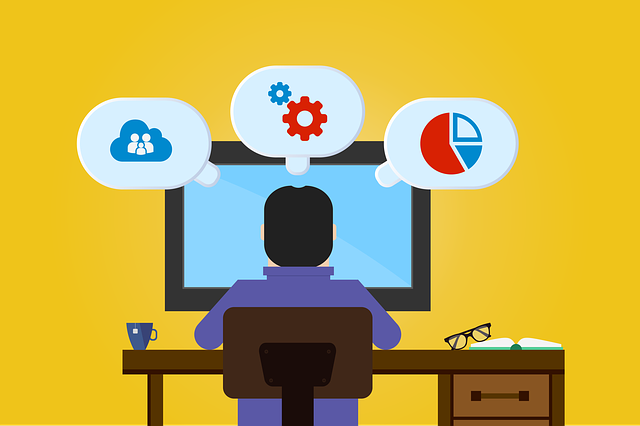
Binds
function.prototype.bind is a method you can use to change the this of the function:
let car = ‘Volvo’
function whatCar() {
console.log(this.car)
};
whatCar(); // outputs ‘Volvo’
whatCar.bind({car: ‘Nissan’})() // outputs ‘Nissan’
whatCar prints the value depending on the assigned this.
But an arrow function doesn’t work with function.prototype.bind because it doesn’t have a local thisBinding. So it just looks at the outer environments this:
let car = ‘Volvo’
let whatCar = () => {
console.log(this.car)
};
whatCar(); // outputs ‘Volvo’
whatCar.bind({car: ‘Nissan’})() // outputs ‘Volvo
Constructor
Normal function can be constructible and callable and arrow function is only callable and not constructible, they can never be invoked with the new keyword:
function hello () {};
let hi = () => {};
new hello(); // works
hi(); // outputs hi is not a constructor
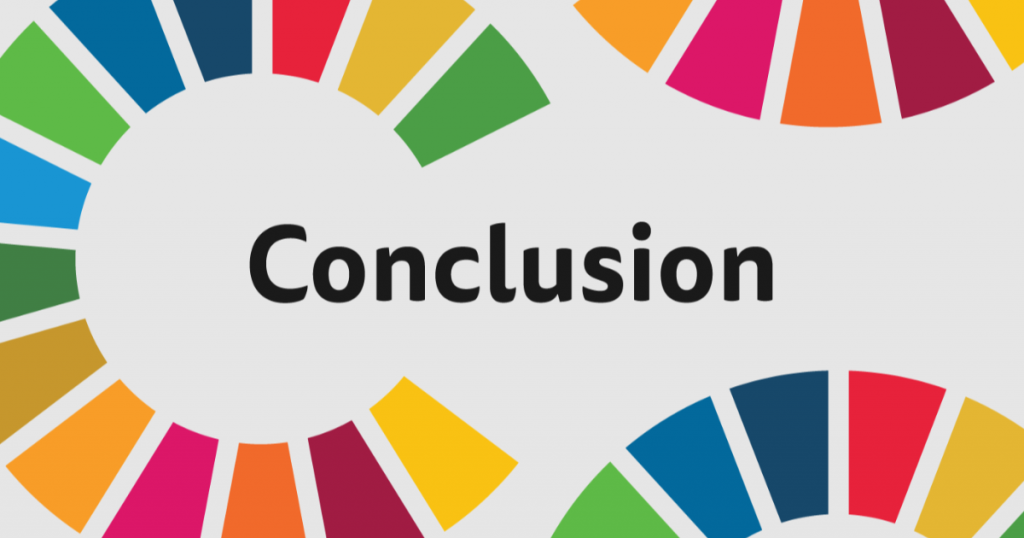
Arrow functions are more handy and stylish, but there are differences between arrow function and normal function. Maybe an arrow function won’t always be the best option to use. All I want to say is the best way to choose what function to use depends on each situation.