Most if not all of the todays’ applications expose some API for interaction. Either for customers or other applications. Application programming interface or API is a software mediator that allows two applications. Each time when we are using Facebook, YouTube or some other app, essentially we are using an API. API is a set of HTTP endpoints that use to send and retrieve data in some form, JSON or XML. Making sure those HTTP endpoints are sending and retrieving correct data thus are working according to the specifications is a vital requirement. Testing APIs belongs to the last (E2E) layer of the testing pyramid for which you may find more information in my previous blog.
Introduction to Rest Assured
Rest Assured is an open-source Java library that is used for testing RESTfull web services. It allows us to write tests using the BDD pattern. Rest Assured is a headless client for accessing Rest web services. The library is highly customizable, allowing us to create a wide variety of request combinations to test different application core business logic combinations.
High customizability also comes in handy when we want to verify the responses from the server, where we can verify the Status code, Status message, Body, Headers, etc. This makes Rest-Assured a versatile library and is often used for API testing.
Rest Assured
Pseudo Syntax:
Given(). param("a", "b"). header("c", "d"). when(). Method(). Then(). statusCode(XXX). body("x, ”y", equalTo("z"));
The syntax of Rest Assured.io is the most interesting part, it’s using the BDD syntax and it’s very understandable.
Explanation:
Code | Description |
Given() | ‘Given’ keyword, is used to set up the (Pre-conditions/ Context), here, you pass the request headers, query and path param, body, cookies. This is optional if these items are not needed in the request |
When() | ‘when’ keyword is a notion that marks the premise of the test |
Method() | Specifies the action of HTTP method (POST,GET,PUT,PATCH,DELETE) |
Then() | Specifies the (Result/Outcomes) and is used for assertions |
Let’s create automation tests
Create new project in intelliJ
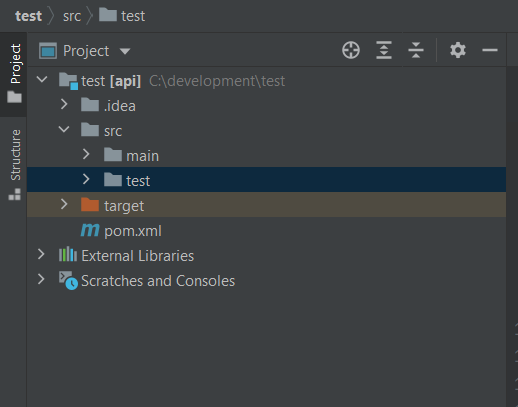
Add the following dependency:
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter</artifactId>
<version>RELEASE</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>io.rest-assured</groupId>
<artifactId>rest-assured</artifactId>
<version>4.4.0</version>
<scope>test</scope>
</dependency>
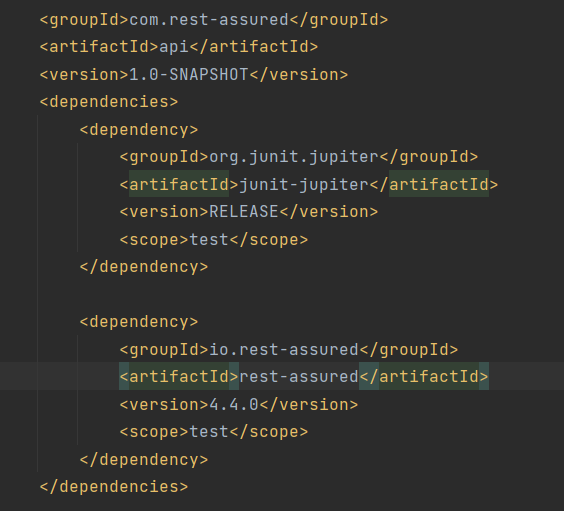
Create new Test class
Simple test example:
public class HelloYouTubeRestAssured {
@Test
public void greetingsYouTube() {
given().when()
.get("http://youtube.com/")
.then()
.statusCode(200);
}
}
The simple test connects to YouTube, performing GET call and making sure that the server responds with a success status code of 200.
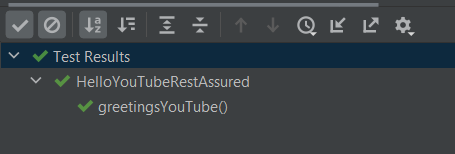
Another tests verifies the Users API:
public class UsersApiTest {
@Test
public void checkUsers() {
given()
.baseUri("https://jsonplaceholder.typicode.com")
.when()
.get("/users")
.then()
.statusCode(200)
.statusLine("HTTP/1.1 200 OK")
.body("id",hasSize(10))
.body("name[0]", equalTo("Leanne Graham"))
.body("username[0]", equalTo("Bret"))
.body("email[0]", equalTo("Sincere@april.biz"))
.body("address[0].city", equalTo("Gwenborough"))
.body("phone[0]", startsWith("1-770-736-8031"))
.body("website[0]", equalTo("hildegard.org"))
.body("company[0].name", equalTo("Romaguera-Crona"));
}
}
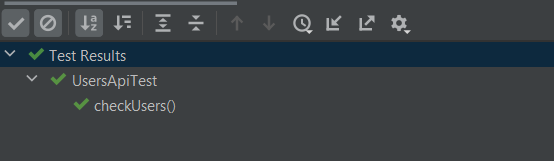
As we can see from the above examples the tests are enclosed in the sense that a single call is performed to the server and only a single response is evaluated. The above test navigates to the Users API of the application and then verifies the response from the server. The verification first verifies that the status code from the code is OK. Then we verify that the response has 10 items after that we verify that the first item has the corresponding data. We are able to assert also inner data of the user object in address[0].city and company[0].name. The assertions which we use are from org.hamcrest which are incorporated into Rest-Assured.
Conclusion
Even though here we have scratched the surface, I hope that you now have a better understanding of Rest-Assured. You can find a working example with the tests on this repository.
Also, you can find more about the Rest-assured usage here.