Vue3 is officially out since September 18 and along with it comes the composition API which is replacing the old options API. The new composition is defined as a set of additive, function-based APIs that allow the flexible composition of component logic.
We are introduced to the composition API because with the old options API as the component grows the readability of the components was not quite pleasant or comfortable and was starting to get messy, the code reuse patterns had some drawbacks, the support for TypeScript was limited etc.
The visual comparison of both APIs look something like this:
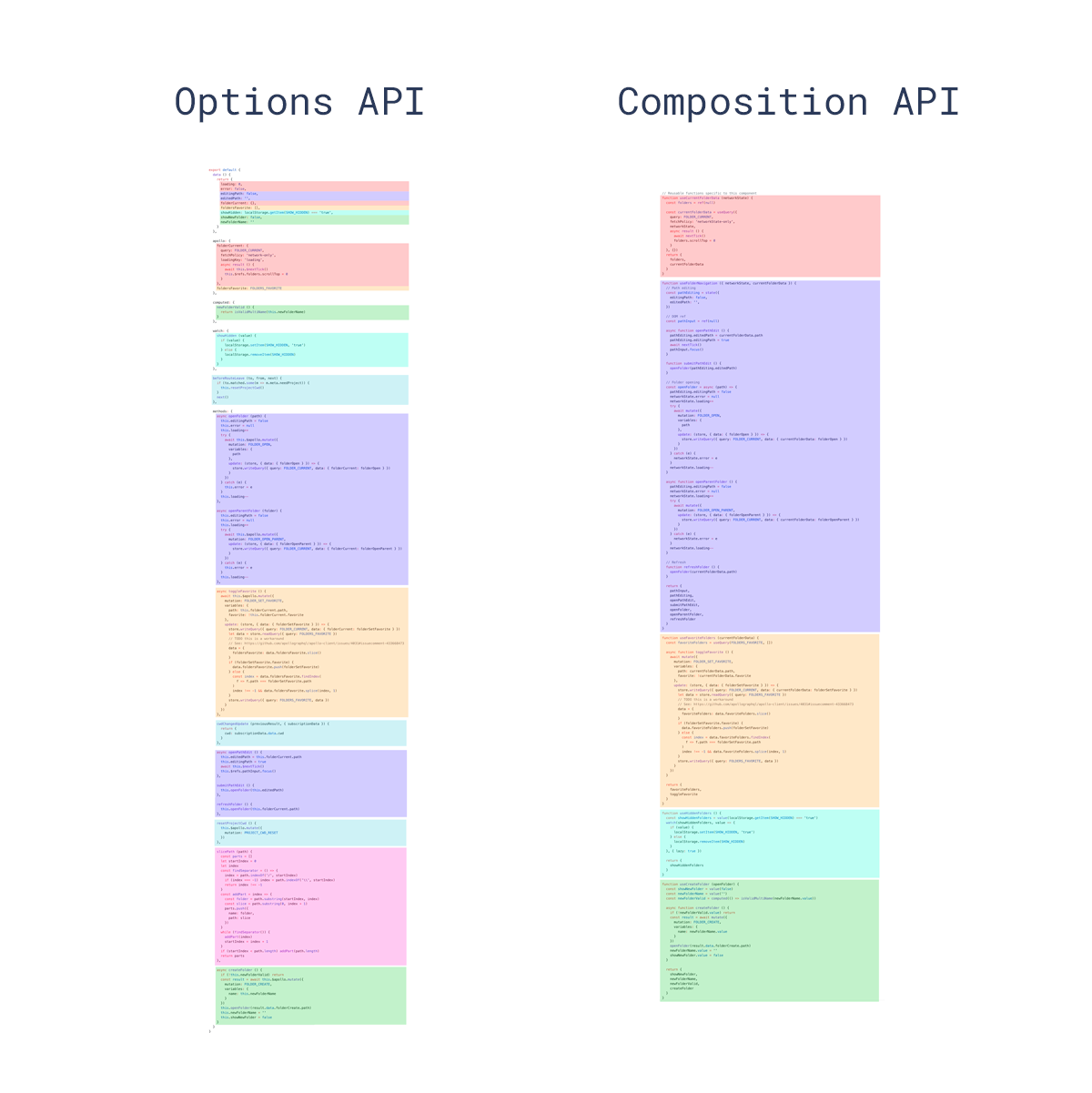
First, we must install @vue/composition-api
via Vue.use()
before using other APIs.
Import the VueCompositionApi:
import Vue from 'vue'
import VueCompositionApi from '@vue/composition-api'
Register the plugin:
Vue.use(VueCompositionApi)
And then we are ready to start.
So, the new API must contain the setup()
function which serves as the entry point for using the composition API inside the components. setup()
is called before the beforeCreate
hook. The component would look like this:
<template>
<div> {{ note }} </div>
<div> {{ data.count }} </div>
</template>
<script>
import { ref, computed, reactive, onMounted } from '@vue/composition-api'
export default {
setup() {
const notes = ref([])
async function getData() {
notes.value = await DataService.getNotesData()
}
const data = reactive({
count: 0,
actions: ['firstAction', 'secondAction', 'thirdAction'],
object: {
foo: 'bar'
}
})
const computedData = computed(() => notes.value)
onMounted(() => {
getData()
}
return {
...toRefs(data),
notes,
computedData
}
}
}
</script>
In the code above we can see the structure of the new API. We have the setup()
function, which is exported in the script tag.
Inside the setup
function we can see several familiar properties.
const notes = ref([])
This is initializing a property inside the setup
function scope. On this property, we must add ref
if we want to make it reactive, which means if we don’t add the ref
and we make a change to that variable, the change won’t be reflected in the DOM. This is the same as initializing variable in the data()
in Vue2:
data() {
return {
notes: []
}
}
As we can see we do not have the method section for creating functions, instead we define the functions in the setup()
. After that, the method is used in the mounted hook which is a bit different than the one in the options API.
Some of the hooks were removed, but almost all of them are available in a similar form.
-> usebeforeCreate
setup()
-> usecreated
setup()
beforeMount
->onBeforeMount
mounted
->onMounted
beforeUpdate
->onBeforeUpdate
updated
->onUpdated
beforeDestroy
->onBeforeUnmount
destroyed
->onUnmounted
activated
->onActivated
deactivated
->onDeactivated
errorCaptured
->onErrorCaptured
In addition, two new debug hooks were added to the composition API:
onRenderTracked
onRenderTriggered
Computed and watch are still available. In the code above we can see how computed is used to return the notes
values.
Reactive()
is similar to ref()
and if we want to create a reactive object we can still use ref()
. But underneath the hood, it’s just calling reactive()
. On the other side, reactive()
will not work with primitive values. It takes an object and returns a reactive proxy of the original. The big difference is when you want to access data defined using reactive()
, for example, if we want to use the count, we must do it like:
<div> {{ data.count }} </div>
One very important thing is to convert a reactive object to plain object is using the toRefs
and return the data like in the component:
return {
...toRefs(data)
}
This is just a small piece of the cake, only something to begin with using the composition API in Vue2 application. For more, you can visit the documentation on the following link.